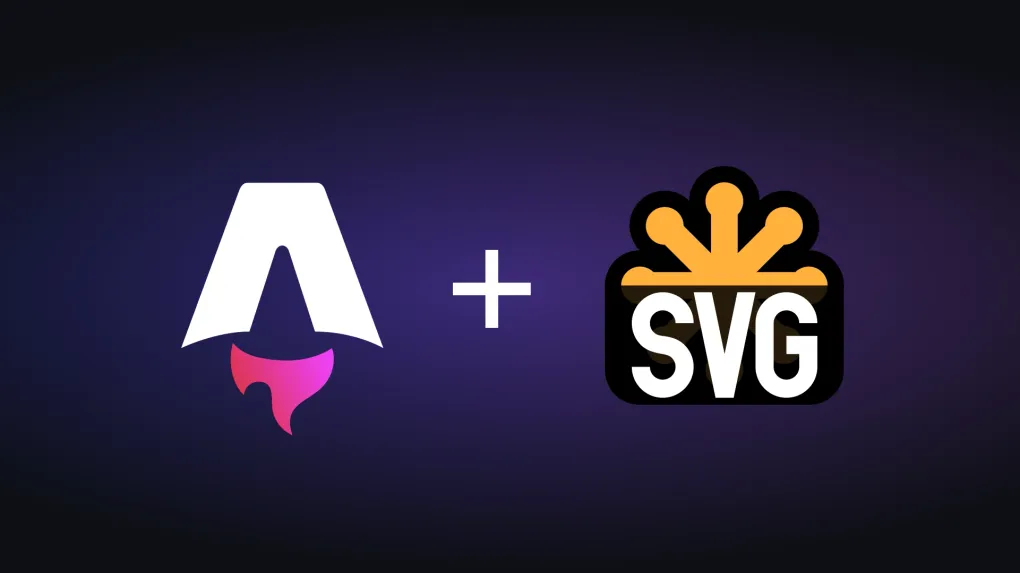
SVG import in Astro
- Astro
If you came to Astro from React world you could be tempted to import your SVG icons like this, especially if you want to use them with Tailwind CSS
---
const Icon from "icons/myicon.svg"
---
<Icon class="w-6 h-6 fill-current text-blue-500">
Sadly, this very convenient method, that we are so used to, will not work.
But Astro is very much capable to give us what we want without to much of a hustle.
First of all we need to import icon as raw
.
---
const Icon from "icons/myicon.svg?raw"
---
Now that we have our svg we need to add a class
on it. Our Icon
constant contains raw svg test as a string,
so first thing that comes to mind is something like this
---
const Icon from "icons/myicon.svg?raw"
const IconWithClass = Icon.replace("<svg", '<svg class="w-6 h-6 fill-current text-blue-500"')
---
This method will definitely work, but what if we will need some more attributes at some point.
The solution is quite simle - a small custom component that will handle any attributes for us.
---
const { raw, ...rest } = Astro.props
const attributesString = Object.entries(rest)
.map(([key, value]) => `${key}="${value}"`)
.join(" ")
---
<Fragment set:html={raw.replace("<svg", `<svg ${attributesString}`)} />
This 7 or so lines of code saved me a lot of time and possible headaches with additional third-party plugins/libraries/components. Use it now, thank me later.